Java, Selenium Exceptions Handle, 셀레니움 예외 처리
<출처> https://www.softwaretestinghelp.com/exception-handling-framework-selenium-tutorial-19/
What is an Exception?
Exceptions are events due to which java program ends abruptly without giving expected output. Java provides a framework where a user can handle exceptions.
The process of handling Exceptions is called Exception Handling.
Exceptions need to be handled because they break the normal flow of execution of a program. One of the important intentions of exception handling is to prevent this break and continue program execution. Sometimes, you might want to perform some series of actions on occurring of a certain exception.
When an exception occurs, an exception object is created which is technically referred to as ‘Throwing an Exception’ and we add Try/Catch blocks like,
try {
// Protected code
} catch (ExceptionName e) {
// Catch block
}
|
#1) The piece of code which might throw an exception is added inside the Try block.
#2) The Catch statement catches the exception and takes it as a parameter.
#3) When no exception is thrown, the try statement is executed and not the catch statement.
Example: When selenium script fails due to the wrong locator, then the developer should be able to understand the reason for failure and this can be achieved easily if the exception is handled properly in the program.
In my experience, it is best to try to avoid WebDriver exceptions whenever possible and catch truly exceptional cases. Use try/catch to handle things that go wrong and are outside my control.
Avoid the ones I can Catch others!
This is the best strategy that has worked for me.
For example, consider a test page that takes more than usual time to load on a test server. We will get frequent exceptions while doing actions on this page. So, instead of just catching this every time, we can
- Add a wait command and try to avoid an exception
- Use ‘Try/Catch’ to handle in case if a truly exceptional case has occurred
Thereby reducing the chances for exceptions.
Advantages and Disadvantages of Avoid-Handle approach
1) This approach reduces the chances of getting exceptions. | 1) Increases the lines of codes because you add extra code to avoid exceptions |
If an exception is still caught it 2) would be a truly exceptional case which is worth checking | 2) Should have a better understanding of Web Driver API, commands and exceptions |
Reduce debugging time. Automation code is intended to find bugs and you don’t want to see too many unwanted 3) exceptions and find the reasons behind each of them | |
4) In the Catch block, you deal with more valid cases | |
5) Reduce false failures | |
6) Clearer report |
In this tutorial, we will discuss Avoid-And-Handle approach for the 10 most common exceptions in Selenium WebDriver. Before that, let’s get a basic understanding of Exception Handling and Try/Catch blocks.
Types of Exceptions in Java and Selenium
Below we have described the types of exceptions and the different ways how we can use exception handling framework in selenium scripts.
There are three kinds of exceptions:
- Checked Exception
- Unchecked Exception
- Error
The class hierarchy of exception and error:
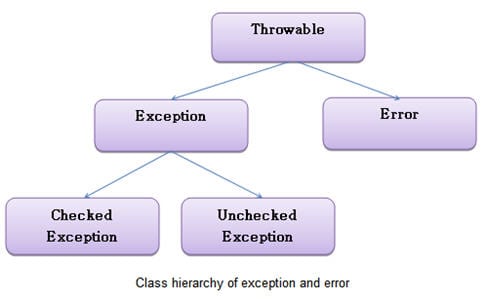
#1) Checked Exception: Checked exception is handled during compile time and it gives the compilation error if it is not caught and handled during compile time.
Example: FileNotFoundException, IOException etc.
#2) Unchecked Exception: In case of the unchecked exception, a compiler does not mandate to handle. The compiler ignores during compile time.
Example: ArrayIndexoutOfBoundException
#3) Error: When a scenario is fatal and the program cannot recover then JVM throws an error. Errors cannot be handled by the try-catch block. Even if the user tries to handle the error by using Try catch block, it cannot recover from the error.
Example: Assertion error, OutOfMemoryError etc.
Exception Handling
Try and Catch block:
try-catch blocks are generally used to handle exceptions. Type of exceptions is declared in catch block which is expected to come. When an exception comes in try block, immediately control moves to catch block.
Example:
try {
br = new BufferedReader(new FileReader("Data"));
} catch(IOException ie)
{
ie.printStackTrace();
}
|
There can be multiple catch blocks for one try block depending upon the type of exception.
Example:
try {
br = new BufferedReader(new FileReader("Data"));
} catch(IOException ie)
{
ie.printStackTrace();
} catch(FileNotFoundException file){
file.printStackTrace();
}
|
throws Exception:
throws keyword in java is used to throw an exception rather than handling it. All checked exceptions can be thrown by methods.
Example:
public static void main(String[] args) throws IOException
{
BufferedReader br=new BufferedReader(new FileReader("Data"));
while ((line = br.readLine()) != null)
{
System.out.println(line);
}
}
|
finally block:
finally, block executes irrespective of execution of try-catch block and it executes immediately after try/catch block completes.
Basically file close, database connection etc. can be closed in finally block.
Example:
try {
br = new BufferedReader(new FileReader("Data"));
} catch(IOException ie)
{
ie.printStackTrace();
}
Finally {
br.close();
}
|
In the above example, BufferReader stream is closed in finally block. br.close() will always execute irrespective of execution of try and catch block.
Note: finally block can exist without any catch block. It is not necessary to have a catch block always.
There can be many catch blocks but only one finally block can be used.
Throwable: Throwable is a parent class for error and exception. Generally, it is difficult to handle errors in java. If a programmer is not sure about the type of error and exception, then it is advised to use the Throwable class which can catch both error and exception.
Example:
try {
br = new BufferedReader(new FileReader("Data"));
} catch (Throwable t)
{
t.printStackTrace();
}
|
Common Exceptions in Selenium WebDriver
Selenium has its own set of exceptions. While developing selenium scripts, a programmer has to handle or throw those exceptions.
Below are a few examples of exceptions in selenium:
All runtime exception classes in Selenium WebDriver come under the superclass WebDriverException.
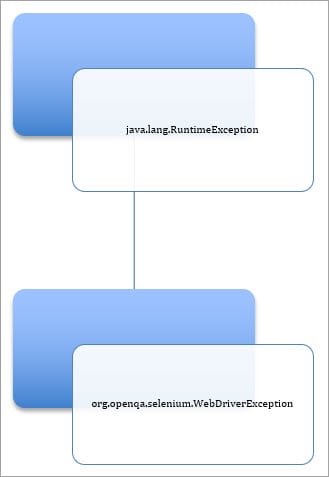
Though there are many Exception classes under WebDriverException, we commonly see the below ones.
- NoSuchElementException
- NoSuchWindowException
- NoSuchFrameException
- NoAlertPresentException
- InvalidSelectorException
- ElementNotVisibleException
- ElementNotSelectableException
- TimeoutException
- NoSuchSessionException
- StaleElementReferenceException
Details:
ElementNotVisibleException: If selenium tries to find an element but the element is not visible within the page
NoAlertPresentException: If a user tries to handle an alert box but the alert is not present.
NoSuchAttributeException: While trying to get attribute value but the attribute is not available in DOM.
NoSuchElementException: This exception is due to accessing an element which is not available on the page.
WebDriverException: Exception comes when a code is unable to initialize WebDriver.
Avoiding And Handling Common Exceptions
Let’s discuss Avoid-And-Handle approach for the above-mentioned exceptions:
#1) org.openqa.selenium.NoSuchElementException
This commonly seen exception class is a subclass of NotFoundException class. The exception occurs when WebDriver is unable to find and locate elements.
Usually, this happens when tester writes incorrect element locator in the findElement(By, by) method.
Consider that in the below example, correct id for the text field was ‘firstfield’ but the tester incorrectly mentioned it as ‘fistfield’. In this case, WebDriver cannot locate the element and org.openqa.selenium.NoSuchElementException will be thrown
driver.findElement(By.id("submit")).click();
Exception Handling:
try {
driver.findElement(By.id("submit")).click();
} catch (NoSuchElementException e)
|
In this case, the exception is thrown even if the element is not loaded.
Avoiding-And-Handling: Try giving a wait command.
Example: The wait command below waits 10 seconds for the presence of web element with id ‘submit’. Then it tries to click it. If the element is available but still the click fails, an exception is caught.
Using delayed time is a common practice in test automation to create a pause in between the steps. By adding a Try/Catch we ensure that the program continues even if the wait couldn’t help.
try {
WebDriverWait wait = new WebDriverWait(driver, TimeSpan.FromSeconds(10));
wait.Until(ExpectedConditions.presenceOfElementLocated(By.id("submit")));
try {
driver.findElement(By.id("submit")).click();
} catch (WebDriverException e) {
System.out.println(“An exceptional case.”);
}
} catch (TimeOutException e) {
System.out.println(“WebDriver couldn’t locate the element”);
}
|
#2) org.openqa.selenium.NoSuchWindowException
NoSuchWindowException comes under NotFoundException class. This is thrown when WebDriver tries to switch to an invalid window.
The below code can throw org.openqa.selenium.NoSuchWindowException if the window handle doesn’t exist or is not available to switch.
driver.switchTo().window(handle_1);
Avoiding-And-Handling: We would use window handles to get the set of active windows and then perform actions on the same.
In the example below, for each window handle, driver switch to is executed. Therefore chances of passing a wrong window parameter reduced.
for (String handle : driver.getWindowHandles()) {
try {
driver.switchTo().window(handle);
} catch (NoSuchWindowException e) {
System.out.println(“An exceptional case”);
}
}
|
#3) org.openqa.selenium.NoSuchFrameException
When WebDriver is trying to switch to an invalid frame, NoSuchFrameException under NotFoundException class is thrown.
The below code can throw org.openqa.selenium.NoSuchFrameException if a frame “frame_11” doesn’t exist or is not available.
driver.switchTo().frame(“frame_11”);
Exception Handling:
try {
driver.switchTo().frame("frame_11");
} catch (NoSuchFrameException e)
|
In this case, the exception is thrown even if the frame is not loaded.
Avoiding-And-Handling: Try to give a wait command.
In the example below, WebDriver waits for 10 seconds for the frame to be available. If the frame is available and still there is an exception, then it is caught.
try {
WebDriverWait wait = new WebDriverWait(driver, TimeSpan.FromSeconds(10));
wait.Until(ExpectedConditions.frameToBeAvaliableAndSwitchToIt(frame_11));
try {
driver.switchTo().frame("frame_11");
} catch (WebDriverException e) {
System.out.println(“An exceptional case”);
}
} catch (TimeOutException e) {
System.out.println(“WebDriver couldn’t locate the frame”);
}
|
#4) org.openqa.selenium.NoAlertPresentException
NoAlertPresentException under NotFoundException is thrown when WebDriver tries to switch to an alert, which is not available.
org.openqa.selenium.NoAlertPresentException will be thrown If below automation code calls accept() operation on Alert() class when an alert is not yet on the screen.
driver.switchTo().alert().accept();
Exception Handling:
try {
driver.switchTo().alert().accept();
} catch (NoSuchAlertException e)
|
In this case, the exception is thrown even if the alert is not loaded completely.
Avoiding-And-Handling: Always use explicit or fluent wait for a particular time in all cases where an alert is expected. If the alert is available and still there is an exception, then it is caught.
try {
WebDriverWait wait = new WebDriverWait(driver, TimeSpan.FromSeconds(10));
wait.Until(ExpectedConditions.alertIsPresent());
try {
driver.switchTo().alert().accept();
} catch (NoAlertPresentException e) {
System.out.println(“An exceptional case”);
}
} catch (TimeOutException e)
System.out.println(“WebDriver couldn’t locate the Alert”);
}
|
#5) org.openqa.selenium.InvalidSelectorException
This subclass of NoSuchElementException class occurs when a selector is incorrect or syntactically invalid. This exception occurs commonly when XPATH locator is used.
Consider the below example:
clickXPathButtonAndWait(“//button[@type=’button’][100]”);
This would throw an InvalidSelectorExeption because the XPATH syntax is incorrect.
Avoiding and Handling: To avoid this, we should check the locator used because the locator is likely incorrect or the syntax is wrong. Using Firebug to find xpath can reduce this exception.
Below code shows how to handle it using Try/Catch
try {
clickXPathButtonAndWait("//button[@type='button']");
} catch (InvalidSelectorException e) {
}
|
#6) org.openqa.selenium.ElementNotVisibleException
ElementNotVisibleException class is a subclass of ElementNotInteractableException class. This exception is thrown when WebDriver tries to perform an action on an invisible web element, which cannot be interacted with. That is, the web element is in a hidden state.
For example, in the below code, if the type of button with id ‘submit’ is ‘hidden’ in HTML, org.openqa.selenium.ElementNotVisibleException will be thrown.
driver.findElement(By.id("submit")).click();
Exception Handling:
try {
driver.findElement(By.id("submit")).click();
} catch (ElementNotVisibleException e)
|
In this case, the exception is thrown even if the page has not loaded completely.
Avoiding-And-Handling: There are two ways to do this. We can either use wait for the element to get completely.
The below code waits 10 seconds for the element. If the element is visible and still exception is thrown, it is caught.
try {
WebDriverWait wait = new WebDriverWait(driver, TimeSpan.FromSeconds(10));
wait.Until(ExpectedConditions.visibilityOfElementLocated(By.id(”submit”));
try {
driver.findElement(By.id("submit")).click();
} catch (WebDriverException e) {
System.out.println(“Exceptional case”);
}
} catch (TimeOutException e)
System.out.println(“WebDriver couldn’t find this element visible”);
}
|
#7) org.openqa.selenium.ElementNotSelectableException
This exception comes under InvalidElementStateException class. ElementNotSelectableException indicates that the web element is present in the web page but cannot be selected.
For example, the below code can throw a ElementNotSelectableException if the id “swift” is disabled.
Select dropdown = new Select(driver.findElement(By.id(“swift”)));
Exception Handling:
try {
Select dropdown = new Select(driver.findElement(By.id(“swift”)));
} catch (ElementNotSelectableException e)
In this case, exception is thrown even if the element becomes enabled after a while.
Avoiding-And-Handling: We can add a wait command to wait until the element becomes clickable. If there is still an exception, it is caught.
try {
WebDriverWait wait = new WebDriverWait(driver, TimeSpan.FromSeconds(10));
wait.Until(ExpectedConditions. elementToBeClickable(By.id(”swift”));
try {
Select dropdown = new Select(driver.findElement(By.id("swift")));
} catch (WebDriverException e) {
System.out.println(“Exceptional case”);
}
} catch (TimeOutException e)
System.out.println(“WebDriver found that this element was not selectable.”);
}
|
#8) org.openqa.selenium.TimeoutException
This exception occurs when a command completion takes more than the wait time. Waits are mainly used in WebDriver to avoid the exception ElementNotVisibleException.
Sometimes test page might not load completely before next command in the program. If WebDriver tries to find an element in the webpage before the page completely loads, then exception ElementNotVisibleException is thrown. To avoid this exception, waits commands are added.
However, if the components don’t load even after the wait, the exception org.openqa.selenium.TimeoutException will be thrown.
driver.manage().timeouts().implicitlyWait(10,TimeUnit.SECONDS) ;
driver.get(“https://www.softwaretestinghelp.com” );
In the above program, an implicit wait of 10 seconds is added. If the page www.softwaretestinghelp.com doesn’t load in 10 seconds, then TimeoutException will be thrown.
Avoiding and Handling: To avoid this, we can manually check the average time for a page to load and adjust the wait
Or, we can add explicit wait using JavaScript executor until the page is loaded.
In the below example, JavaScript executor is used. After page navigation, we call JavaScript return document.readyState for 20 seconds until “complete” is returned.
WebDriverWait wait = new WebDriverWait(driver, TimeSpan.FromSeconds(30));
wait.until(webDriver -> ((JavascriptExecutor)webDriver).executeScript("return document.readyState").equals("complete"));
driver.get("https://www.softwaretestinghelp.com");
|
#9) org.openqa.selenium.NoSuchSessionException
This exception is thrown when a method is called after quitting the browser by WebDriver.quit(). This can also happen due to web browser issues like crashes and WebDriver cannot execute any command using the driver instance.
To see this exception, the code below can be executed.
driver.quit()
Select dropdown = new Select(driver.findElement(By.id(“swift”)));
Avoiding and Handling: Always choose the latest stable version of browser to run Selenium Webdriver testcases.
This exception can be reduced by using driver.quit() at the completion of all tests. Do not try to use them after each test case. This can lead to issues when driver instance is null and upcoming test cases try to use it without initializing.
The below code creates WebDriver instance in the @BeforeSuite TestiNG annotation and destroys it in @AfterSuite TestiNG annotation
@BeforeSuite
public void setUp() throws MalformedURLException {
WebDriver driver = new FirefoxDriver();
}
@AfterSuite
public void testDown() {
driver.quit();
}
|
#10) org.openqa.selenium.StaleElementReferenceException
This exception says that a web element is no longer present in the web page.
This error is not the same as ElementNotVisibleException.
StaleElementReferenceException is thrown when an object for a particular web element was created in the program without any problem and however; this element is no longer present in the window. This can happen if there was a
- Navigation to another page
- DOM has refreshed
- A frame or window switch
WebElement firstName = driver.findElement(By.id(“firstname”));
driver.switchTo().window(Child_Window);
element.sendKeys(“Aaron”);
In the code above, object firstName was created and then the window was switched. Then, WebDriver tries to type ‘Aaron’ in the form field. In this case StaleElementReferenceException is thrown.
Avoiding and Handling: Confirm that we are trying to do the action in the correct window. To avoid issues due to DOM refresh, we can use Dynamic Xpath
Let’s discuss another example.
Say ‘id’ of a username field is ‘username_1’ and the XPath will be //*[@id=’firstname_1?]. When you open the page again the ‘id’ might change say to ‘’firstname _11’. In this case, the test will fail because the WebDriver could not find the element. In this case, StaleElementReferenceException will be thrown.
In this case, we can use a dynamic xpath like,
try {
driver.findElement(By.xpath(“//*[contains(@id,firstname’)]”)).sendKeys(“Aaron”);
} catch (StaleElementReferenceException e)
|
In the example above dynamic XPATH is used and if the exception is still found, it is caught.
Conclusion
Exception handling is the essential part of every java program as well as selenium script. We can build robust and optimal code by handling an exception in smart ways. And it is also a best practice to handle exceptions in a script which will give you a better report when a program fails due to any reason.
Here we have tried to cover the process and framework of exception handling which is required to be implemented in selenium scripts.
Remember it is not mandatory to always handle the exception in a try-catch block. You can also throw an exception depending upon the requirement in a script.
An exception shouldn’t be ignored as they break program execution. In this tutorial, we went through different exceptions and ways to reduce the chances of getting them through manual checks and codes.
Adding waits can control some cases like ‘NoSuchElementException‘, ‘ElementNotFoundException‘, ‘ElementNotVisibleException‘.
Next Tutorial #20: In the upcoming tutorial, we would discuss the various types of testing frameworks available. We would also study the pros and cons of using a fledged framework approach in automation testing. We would discuss in detail about the Test data-driven framework.
Please post your queries, related to handling exception in Selenium WebDriver, if you have any.
Recommended Reading
'웹 크롤링, 스크래핑' 카테고리의 다른 글
셀레니움 자바 vs. 파이썬 함수(메서드) 비교 (0) | 2022.07.03 |
---|---|
Beautiful Soup 공식 레퍼런스 사이트 (0) | 2022.07.02 |
Selenium, 링크나 버튼을 클릭할 때 click() 함수 반응이 없는 경우 (0) | 2022.06.22 |
셀레니움, User-Agent 변경하여 접속하기 (0) | 2022.06.21 |
셀레니움 쿠팡 로그인 차단 접속 거부 Access Denied 해결 (0) | 2022.06.21 |
32 thoughts on “Top 10 Selenium Exceptions and How To Handle These (Exact Code)”
Can we have a session on fitnesse+selenium+Eclipse . Please
ReplyThanks
In try and catch block if error occurred then it will be handle in catch block but what about happen if we can used throws ?
ReplyHi very useful.
ReplyCan you share some simple usage examples of sleneium exceptions mentioned in the end?
Hi Keyur,throws will be used when you dont want to handle any error.Lets say you are you want to find an element in webpage but the element is not shown for some reason.In this case you may not want your code to fail because element is not available.So in this case you can catch the exception and navigate again to the elemnet.If you want to fail the testcase in first time if you dont find the element then use throwd.It will come out of the programme.
ReplySupriya.Hope this example solves your doubt.
public class elementNotfoundExample {
public static void main(String[] args) throws InterruptedException {
WebDriver driver=new FirefoxDriver();
try{
driver.findElement(By.xpath(“//*[@id=’username’]”));
}catch(ElementNotFoundException e)
{
Thread.sleep(10);
driver.findElement(By.xpath(“//*[@id=’username’]”));
}
}
}
Replyif the element (By.xpath(“//*[@id=’username’]”) cames after 10 seconds
Replyyou should use wait.until function with try.
Hi,
I am currently working on a project in which I have created scripts using Web Driver & TestNG Reporting.
I have put the code for all the main test scripts under try…catch block to handle exceptions. After running the test suite I am able to successfully catch the exceptions in eclipse console. But the problem I am facing is “Results of running suite” (TestNG) tab in eclipse shows all the scrips as passed.
I am looking for such a mechanism so that if any exception occurs while executing the test suite it should mark the corresponding test/method as failed.
Please suggest.
Replygive a soft assertion, it will mark the tests if they
Replyare failed
Hi,
am using the below files
iedriver-2.46-32bit—-browser version ie-11
chromedriver-2.16——browser version 44.0
standalone server-2.46.0
i am getting the below issues
–>just i able to login my application after then i need to click on element by using mousehover actions
but its not executing properly.
–>if i execute multiple times mouse hover action is not executing in chrome browser
–>In ie browser its executing instead of one element its performing action on another element.
how can i resolve these issues
can anyone suggest me please…
Thanks & Regards,
ReplyPrasanthi
Can we automate deskdop applications using selenium web driver.
ReplyNo we need to use winium desktop driver. rest the process is similar to selenium like driver.findelement(by.id(“dummy”)).click etc…..
ReplyNo it’ll not support however you can use QTP tool, its a paid version.
ReplyThis is useful context, but it would be nice if you mention differences between Throws,Throw,Throwable classes
ReplyUseful context. Thanks.
ReplyTo answer your question Vidya, no Selenium cannot be used to automate desktop applications. For that you could look at AutoHotkey which is free. There are other utilities such as AutoIt, but I don’t know if they are free or not.
Replyhi vidya,prasanthi i need to learn selnium pls help
Replyi hate selenium
Replyi want to learn pls help
ReplyI want to upgrade
Replypackage demo1;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class writeexcelsheet {
public static void main(String[] args) throws IOException {
// TODO Auto-generated method stub
FileInputStream file= new FileInputStream(“D:\\Book1.xlsx”);
Workbook wob =new XSSFWorkbook(file);
Sheet wos=wob.getSheet(“logindata”);
Row rowcount;
rowcount=wos.getRow(1);
Cell col;
col= rowcount.getCell(2);
col.setCellValue(“pass”);
Row rowcount1;
rowcount1=wos.getRow(2);
Cell col1;
col1= rowcount1.getCell(2);
col1.setCellValue(“fail”);
FileOutputStream fileo= new FileOutputStream(“D:\\Book1.xlsx”);
wob.write(fileo);
wob.close();
}
}
Replyabove program when execute getting error:
exception thred “main” java.lang.nullpointerexception
please help me.
Points to me noted:
1. You are handling the IOException wrong way.
2. While you are reading or writing to the Excel file you are closing the Excel Workbook I/O Streams
Here how it should be done:
package com.datadriven;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class WriteToExcel {
private static FileInputStream fis;
private static FileOutputStream fos;
private static XSSFWorkbook workbook;
private static XSSFSheet sheet;
public static void main(String[] args) {
try {
fileInput(“D:”, “Book1.xlsx”);
workbook = new XSSFWorkbook(fis);
sheet = workbook.getSheet(“logindata”);
Row rowcount;
rowcount = sheet.getRow(1);
Cell col;
col = rowcount.getCell(2);
col.setCellValue(“pass”);
Row rowcount1;
rowcount1 = sheet.getRow(2);
Cell col1;
col1 = rowcount1.getCell(2);
col1.setCellValue(“fail”);
fos = fileOutput(“D:”, “Book1.xlsx”);
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
fis.close();
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
public static void fileInput(String path, String fileName) {
try {
fis = new FileInputStream(path + “\\” + fileName);
} catch (IOException e) {
e.printStackTrace();
}
}
public static FileOutputStream fileOutput(String path, String fileName) {
Replytry {
fos = new FileOutputStream(path + “\\” + fileName);
workbook.write(fos);
} catch (IOException e) {
e.printStackTrace();
}
return fos;
}
}
Exception is an error event that can happen during the execution of a program and disrupts its normal flow. Java provides a robust and object oriented way to handle exception scenarios, known as Java Exception Handling. Many Thanks for sharing this blog.
ReplyI appreciate the fact that so many folks do write-ups on this topic, however they don’t seem to be real life examples. By that I mean the following:
1. Scripts are run via something like jenkins, I don’t think its practical to do system.out.println(s), a logger would probably be used.
2. We are going to use a framework like testng/junit. where you have to fail the test on an exception.
3. test scripts typically have multiple steps, we can’t really put individual try/catch(s) around each step.
4. generally folks are using the PageObject model, so how do exceptions get handled if they occur on a page object.
5. What kind of information can i get from these exceptions? Like is there a way to get the locator used?
For me I’d much rather see how Exception(s) are handled under the above circumstances.
ReplyHi All,
I want to get mail whenever exception occurs in script or even if I get a error in between script while execution is in Progress , Please guide me how can I implement this.( in selenium testing using Java)
Regards,
ReplyAbhi
Hello,
Problem :In my application, there is the unexpected popup shows up on any screen that makes test case fail. I can surround findelement with try-catch for most likely places but it may happen on any screen so this is not a good idea to cover every finelement statement with try-catch.
Approach : I would like to have mechanism if findlement is failed at any place due to unexpected pop up occurred, Framework should handle pop up and retry same find element statement one more time and return back to test execution to continue future steps.
After doing some research on Internet, I feel it can be done using WebDriverEventListener and tried but did not work.
Solution/Suggestion: Can someone please provide me solution or suggestion how this can be achieved.
I am using Java Selenium TestNG in Framework
Thank you
ReplyThe main difference between checked and unchecked exception is that the checked exceptions are checked at compile-time while unchecked exceptions are checked at runtime. Thanks for sharing this article.
ReplyWhat about stale element exception ?
Replyfor(int i=0; i<LoginA.username1.length; i++)
{
Thread.sleep(2000);
driver.findElement(By.id("imglogin")).click();
Thread.sleep(2000);
driver.findElement(By.id("Login_txtusername")).clear();
driver.findElement(By.id("Login_txtusername")).sendKeys(LoginA.username1[i]);
System.out.println(LoginA.username1[i]);
driver.findElement(By.id("Login_txtpassword")).clear();
driver.findElement(By.id("Login_txtpassword")).sendKeys(LoginA.password1[i]);
System.out.println(LoginA.password1[i]);
//Click on login button
driver.findElement(By.id("Login_btnlogin")).click();
// Click on Ok button
driver.findElement(By.className("confirm")).click();
Thread.sleep(2000);
driver.findElement(By.id("imglogin")).click();
Thread.sleep(2000);
driver.findElement(By.id("Login_imglogot")).click();
Thread.sleep(2000);
if(LoginA.username1[i]=="garima" && LoginA.password1[i]=="123")
{
//Scroll-down page by JavaScript
WebElement doctor = driver.findElement(By.id("imgDoctor"));
js.executeScript("arguments[0].scrollIntoView(true);" , doctor);
Thread.sleep(2000);
driver.findElement(By.id("imgDoctor")).click();
}
}